Assessment: Way of the Programmer Week Four >> Python Basics
seqmut-1-1: Which of these is a correct reference diagram following the execution of the following code?
lst = ['mercury', 'venus', 'earth', 'mars', 'jupiter', 'saturn', 'uranus', 'neptune', 'pluto']
lst.remove('pluto')
first_three = lst[:3]
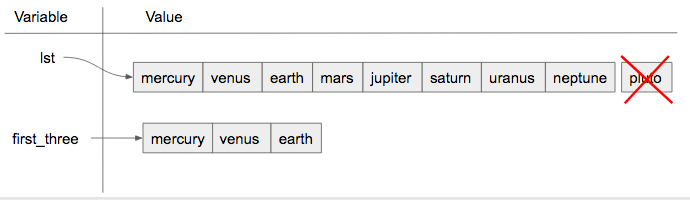
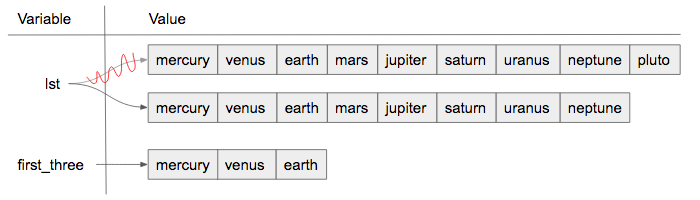
Multiple Choice (assess_question4_1_1_1)
Score: 1.0 / 1
seqmut-1-4: What will be the value of a
after the following code has executed?
a = ["holiday", "celebrate!"]
quiet = a
quiet.append("company")
The value of a
will be
Good work!
Fill in the Blank (assess_question3_3_1_1)
Score: 1.0 / 1
seqmut-1-5: Could aliasing cause potential confusion in this problem?
b = ['q', 'u', 'i']
z = b
b[1] = 'i'
z.remove('i')
print(z)
Multiple Choice (assess_question3_3_1_2)
Score: 1.0 / 1
seqmut-1-13: Given that we want to accumulate the total sum of a list of numbers, which of the following accumulator patterns would be appropriate?
nums = [4, 5, 2, 93, 3, 5]
s = 0
for n in nums:
s = s + 1
nums = [4, 5, 2, 93, 3, 5]
s = 0
for n in nums:
s = n + n
nums = [4, 5, 2, 93, 3, 5]
s = 0
for n in nums:
s = s + n
Multiple Choice (assess_question5_2_1_1)
Score: 1.0 / 1
seqmut-1-14: Given that we want to accumulate the total number of strings in the list, which of the following accumulator patterns would be appropriate?
lst = ['plan', 'answer', 5, 9.29, 'order, items', [4]]
s = 0
for n in lst:
s = s + n
lst = ['plan', 'answer', 5, 9.29, 'order, items', [4]]
for item in lst:
s = 0
if type(item) == type("string"):
s = s + 1
lst = ['plan', 'answer', 5, 9.29, 'order, items', [4]]
s = ""
for n in lst:
s = s + n
lst = ['plan', 'answer', 5, 9.29, 'order, items', [4]]
s = 0
for item in lst:
if type(item) == type("string"):
s = s + 1
Multiple Choice (assess_question5_2_1_2)
Score: 1.0 / 1
seqmut-1-15: Which of these are good names for an accumulator variable? Select as many as apply.
- Yes, total is a good name for accumulating numbers.
- Yes, accum is a good name. It’s both short and easy to remember.
Multiple Choice (assess_question5_2_1_3)
Score: 1.0 / 1
seqmut-1-16: Which of these are good names for an iterator (loop) variable? Select as many as apply.
- Yes, item can be a good name to use as an iterator variable.
- Yes, elem can be a good name to use as an iterator variable, especially when iterating over lists.
- Yes, char can be a good name to use when iterating over a string, because the iterator variable would be assigned a character each time.
Multiple Choice (assess_question5_2_1_4)
Score: 1.0 / 1
seqmut-1-17: Which of these are good names for a sequence variable? Select as many as apply.
- Yes, num_lst is good for a sequence variable if the value is actually a list of numbers.
- Yes, this is good to use if the for loop is iterating through a string.
- Yes, names is good, assuming that the for loop is iterating through actual names and not something unrelated to names.
Multiple Choice (assess_question5_2_1_5)
Score: 1.0 / 1
seqmut-1-18: Given the following scenario, what are good names for the accumulator variable, iterator variable, and sequence variable? You are writing code that uses a list of sentences and accumulates the total number of sentences that have the word ‘happy’ in them.
Multiple Choice (assess_question5_2_1_6)
Score: 1.0 / 1
For each character in the string saved in ael
, append that character to a list that should be saved in a variable app
.
ael = "python!"
lst = []
for i in ael:
lst.append(i)
app = lst
ActiveCode (access_ac_5_2_1_1)
Result | Actual Value | Expected Value | Notes |
---|---|---|---|
Pass | [‘p’,… ‘!’] | [‘p’,… ‘!’] | Testing that app has the correct elements. |
Pass | ‘append’ | ‘ael =…= lst’ | Testing that your code uses append. |
You passed: 100.0% of the tests
Score: 1.0 / 1
For each string in wrds
, add ‘ed’ to the end of the word (to make the word past tense). Save these past tense words to a list called past_wrds
.
wrds = ["end", 'work', "play", "start", "walk", "look", "open", "rain", "learn", "clean"]
lst = []
for i in wrds:
i = i + "ed"
lst.append(i)
past_wrds = lst
ActiveCode (access_ac_5_2_1_2)
Result | Actual Value | Expected Value | Notes |
---|---|---|---|
Pass | [‘end…ned’] | [‘end…ned’] | Testing that past_wrds has the correct value. |
Pass | ‘for ‘ | ‘wrds …= lst’ | Testing that your code uses a for loop. |
You passed: 100.0% of the tests